12 essential SQL commands for database management
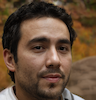

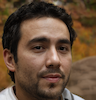
Mastering SQL: 12 Essential Commands for Database Management
SQL (Structured Query Language) is the standart language for managing relational databases. It's used to store, manipulate, and retrieve data stored in a database. As a database administrator, developer, or data analyst, having a solid understanding of SQL commands is crucial for efficient database management. In this article, we'll explore 12 essential SQL commands that will help you master database management.
Creating and Modifying Database Structures
When working with databases, creating and modifying database structures is a fundamental task. Two essential SQL commands for achieving this are CREATE
and ALTER
.
The CREATE
Command
The CREATE
command is used to create a new database, table, index, or view. The basic syntax for creating a table is as follows:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
...
);
For example, to create a table called employees
with three columns – id
, name
, and salary
– you would use the following SQL command:
CREATE TABLE employees (
id INT PRIMARY KEY,
name VARCHAR(255),
salary DECIMAL(10, 2)
);
The ALTER
Command
The ALTER
command is used to modify an existing database structure, such as adding or removing columns from a table. The basic syntax for modifying a table is as follows:
ALTER TABLE table_name
ADD | MODIFY | DROP column_name datatype;
For example, to add a new column called department
to the employees
table, you would use the following SQL command:
ALTER TABLE employees
ADD department VARCHAR(255);
Data Manipulation Language (DML) Commands
DML commands are used to manipulate data in a database. Three essential DML commands are INSERT
, UPDATE
, and DELETE
.
The INSERT
Command
The INSERT
command is used to add new data to a table. The basic syntax for inserting data is as follows:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
For example, to insert a new employee into the employees
table, you would use the following SQL command:
INSERT INTO employees (name, salary, department)
VALUES ('John Doe', 50000.00, 'Sales');
The UPDATE
Command
The UPDATE
command is used to modify existing data in a table. The basic syntax for updating data is as follows:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
For example, to update the salary of an employee in the employees
table, you would use the following SQL command:
UPDATE employees
SET salary = 55000.00
WHERE name = 'Jane Doe';
The DELETE
Command
The DELETE
command is used to delete data from a table. The basic syntax for deleting data is as follows:
DELETE FROM table_name
WHERE condition;
For example, to delete an employee from the employees
table, you would use the following SQL command:
DELETE FROM employees
WHERE name = 'Bob Smith';
Data Control Language (DCL) Commands
DCL commands are used to control access to a database. Two essential DCL commands are GRANT
and REVOKE
.
The GRANT
Command
The GRANT
command is used to give permissions to a user or role to perform certain actions on a database object. The basic syntax for granting permissions is as follows:
GRANT privilege ON table_name TO user_or_role;
For example, to grant the SELECT
privilege on the employees
table to a user called Reporting
, you would use the following SQL command:
GRANT SELECT ON employees TO Reporting;
The REVOKE
Command
The REVOKE
command is used to take away permissions from a user or role. The basic syntax for revoking permissions is as follows:
REVOKE privilege ON table_name FROM user_or_role;
For example, to revoke the INSERT
privilege on the employees
table from a user called HR
, you would use the following SQL command:
REVOKE INSERT ON employees FROM HR;
Querying Data
Querying data is an essential part of database management. Three essential SQL commands for querying data are SELECT
, JOIN
, and SUBQUERY
.
The SELECT
Command
The SELECT
command is used to retrieve data from one or more tables. The basic syntax for selecting data is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
For example, to retrieve the names and salaries of all employees in the employees
table, you would use the following SQL command:
SELECT name, salary
FROM employees;
The JOIN
Command
The JOIN
command is used to combine data from two or more tables based on a common column. The basic syntax for joining tables is as follows:
SELECT column1, column2, ...
FROM table1
JOIN table2
ON table1.column_name = table2.column_name;
For example, to retrieve the names and departments of all employees in the employees
table and their corresponding department names from the departments
table, you would use the following SQL command:
SELECT e.name, d.department_name
FROM employees e
JOIN departments d
ON e.department = d.department_id;
The SUBQUERY
Command
The SUBQUERY
command is used to nest a query inside another query. The basic syntax for using a subquery is as follows:
SELECT column1, column2, ...
FROM table_name
WHERE condition
(SELECT column1, column2, ...
FROM table_name
WHERE condition);
For example, to retrieve the names of all employees who earn a salary higher than the average salary, you would use the following SQL command:
SELECT name
FROM employees
WHERE salary > (
SELECT AVG(salary)
FROM employees
);
Other Essential SQL Commands
In addition to the commands mentioned above, there are several other essential SQL commands that are useful for database management.
The DROP
Command
The DROP
command is used to delete a database object, such as a table or index. The basic syntax for dropping a table is as follows:
DROP TABLE table_name;
The TRUNCATE
Command
The TRUNCATE
command is used to delete all data from a table. The basic syntax for truncating a table is as follows:
TRUNCATE TABLE table_name;
The INDEX
Command
The INDEX
command is used to create an index on a table column. The basic syntax for creating an index is as follows:
CREATE INDEX index_name ON table_name (column_name);
Conclusion
In this article, we've explored 12 essential SQL commands for database management. From creating and modifying database structures to querying data, these commands are crucial for any database administrator, developer, or data analyst. By mastering these commands, you'll be able to efficiently manage and analyze data, making informed business decisions and driving business growth.